Introduction
This book describes how to use the CacheClient .NET assembly, which provides two different but complimentary ways to access Caché from a .NET application:
-
The Caché .NET Object Binding — provides high-performance native object access to data using auto-generated proxy classes. These proxy classes correspond to persistent objects stored within the Caché database and provide object persistence, retrieval, data caching, and life-cycle management (see “Using the Caché Object Binding for .NET”).
-
The Caché implementation of the ADO.NET Managed Provider — provides easy relational access to data using the standard ADO.NET Managed Provider classes (see “Using ADO.NET Managed Provider Classes”).
This combination is unique in that it provides a .NET application with simultaneous relational and object access to data, using a common API and without requiring any object-to-relational mapping. The CacheClient assembly is implemented using .NET managed code throughout, making it easy to deploy within a .NET environment. It is thread-safe and can be used within multithreaded .NET applications.
Installation and Configuration
This section provides specifies requirements and provides instructions for installing Caché and configuring Visual Studio.
Requirements
-
The .NET Framework, versions 2.0, 3.0, 4.0, or 4.5.
-
Caché 5.1 or higher
-
Visual Studio 2008 or 2010. Visual Studio 2010 is required when using .NET 4.0 or 4.5.
Caché is not required on computers that run your Caché .NET client applications, but they must have a TCP/IP connection to the Caché Server and must be running a supported version of the .NET Framework.
Installation
The CacheClient assembly (InterSystems.Data.CacheClient.dll) is installed along with the rest of Caché, and requires no special preparation.
-
When installing Caché in Windows, select the Setup Type: Development option.
-
If Caché has been installed with security level 2, open the Management Portal and go to System Administration > Security > Services, select %Service_CallIn, and make sure the Service Enabled box is checked. If you installed Caché with security level 1 (minimal) it should already be checked.
To use the CacheClient assembly in a .NET project, you must add a reference to the assembly, and add the corresponding Using statements to your code (as described in the following section, “Configuring Visual Studio”).
There is a separate version of InterSystems.Data.CacheClient.dll for each supported version of .NET. In the current release of Caché, these files are located in the following subdirectories of <Cache-install-dir>\dev\dotnet\bin:
-
.NET version 2.0: \bin\v2.0.50727
-
.NET version 3.0: \bin\v3.0
-
.NET version 4.0: \bin\v4.0.30319
-
.NET version 4.5: \bin\v4.5
See “Caché Installation Directory” in the Caché Installation Guide for the location of <Cache-install-dir> on your system.
All Caché assemblies for .NET are installed to the .NET GAC (Global Assembly Cache) when Caché is installed.
Configuring Visual Studio
This chapter describes how to set up a Visual Studio project using the CacheClient assembly, and how to add the Caché Object Binding Wizard to Visual Studio. The following topics are covered:
-
Configuring a Visual Studio Project — describes how to add a CacheClient assembly reference and Using statements.
-
Adding the Caché Object Binding Wizard to Visual Studio — describes how to add the Caché proxy class creation wizard to the Visual Studio Tools menu.
Configuring a Visual Studio Project
To add a CacheClient assembly reference to a project:
-
From the Visual Studio main menu, select Project > Add Reference
-
In the Add Reference window, click on Browse...
-
Browse to the subdirectory of <Cache-install-dir>\dev\dotnet\bin that contains the assembly for the version of .NET used in your project (see “Installation”), select InterSystems.Data.CacheClient.dll, and click OK.
-
In the Visual Studio Solution Explorer, the InterSystems.Data.CacheClient assembly should now be listed under References:
Add Using statements for the two main namespaces in the InterSystems.Data.CacheClient.dll assembly before the beginning of your application's namespace.
using InterSystems.Data.CacheClient;
using InterSystems.Data.CacheTypes;
namespace DotNetSample {
...
}
Both the CacheClient and CacheTypes namespaces are included in the InterSystems.Data.CacheClient.dll assembly.
Adding the Object Binding Wizard to Visual Studio
The Caché Object Binding Wizard is a program to generate Caché proxy objects (see “Using the Caché Object Binding Wizard”). It can be run from the command line, but will be more readily available if you integrate it into Visual Studio by adding it to the External Tools menu.
To add the Caché Object Binding Wizard to the Tools menu:
-
From the Visual Studio main menu, select Tools > External Tools...
-
In the External Tools window:
-
Click Add
-
In the Title field, enter : Cache Object Binding Wizard
-
In the Command field, browse to the <Cache-install-dir>\dev\dotnet\bin\v2.0.50727 directory and select CacheNetWizard.exe. (Although this executable is located in the .NET 2.0 directory, it provides the Binding Wizard for all supported versions of .NET. For the location of <Cache-install-dir> on your system, see “Caché Installation Directory” in the Caché Installation Guide).
-
Click OK
The Caché Object Binding Wizard now will be displayed as an option on the Visual Studio Tools menu.
-
Caché .NET Binding Architecture
The Caché .NET binding gives .NET applications a way to interoperate with objects contained within a Caché server. These objects can be persistent objects stored within the Caché object database or they can be transient objects that perform operations within a Caché server.
The Caché .NET Binding consists of the following components:
-
The Caché Object Server — a high performance server process that manages communication between .NET objects and a Caché database server using standard networking protocols (TCP/IP). Caché uses a common server for .NET, C++, Java, Perl, Python, ODBC, and JDBC access.
-
The InterSystems.Data.CacheClient assembly — a set of .NET classes that implement all the functionality of the .NET classes created by the Caché Proxy Generator. It also provides a set of proxy classes for a few Object Server classes that are projected differently to make them fit into the framework of the .NET standard library.
-
The Caché Proxy Generator — a set of methods that generate .NET classes from classes defined in the Caché Class Dictionary. Several different interfaces are available (see “Generating Caché Proxy Classes”).
The Proxy Generator can create .NET proxy classes for any class in the Caché Class Dictionary. The proxy classes contain only managed .NET code, which the Proxy Generator creates by inspecting the class definitions found in the Caché Class Dictionary. Instances of the .NET proxy classes on the client communicate at runtime (using TCP/IP sockets) with their corresponding Caché objects on a Caché server. This is illustrated in the following diagram:
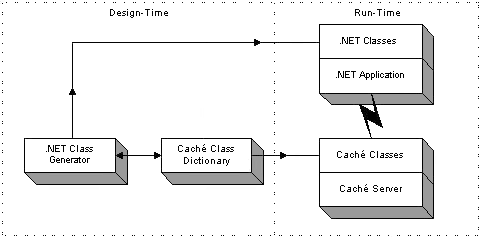
The basic mechanism works as follows:
-
You define one or more classes within Caché. These can be persistent objects stored within the Caché database or transient objects that run within a Caché server.
-
The Caché Proxy Generator creates .NET proxy classes that correspond to your Caché classes. These classes contain stub methods and properties that correspond to Caché object methods and properties on the server.
-
At runtime, your .NET application connects to a Caché server. It can then create instances of .NET proxy objects that correspond to objects within the Caché server. You can use these proxy objects as you would any other .NET objects. Caché automatically manages all communications as well as client-side data caching.
The runtime architecture consists of the following:
-
A Caché database server (or servers).
-
A .NET client application into which your generated and compiled .NET proxy classes have been linked.
At runtime, the .NET application connects to Caché using an object connection interface (provided by the CacheConnection class). All communication between the .NET application and the Caché server uses the standard TCP/IP protocol.
The Caché .NET Help File
The Caché .NET help file provides the most current and detailed documentation for both object and relational APIs. Although the file is named CacheProvider.chm, it covers both ADO.NET Managed Provider classes (InterSystems.Data.CacheClient) and Object Binding classes (InterSystems.Data.CacheClient.ObjBind), as well as classes used by both bindings. CacheProvider.chm is available as a stand-alone help file in <Cache-install-dir>\dev\dotnet\help.
The Caché .NET Sample Programs
Caché comes with a set of sample projects that demonstrate the use of the Caché .NET binding. These samples are located in the <Cache-install-dir>/dev/dotnet/samples/ subdirectory of the Caché installation (see “Caché Installation Directory” in the Caché Installation Guide for the location of <Cache-install-dir> on your system).
-
adoform — A simple program to access and manipulate the Sample.PersonOpens in a new tab database. The same program is presented in three different Visual Studio languages: C#, Basic, and C++.
-
bookdemos — Contains complete, working versions of the examples in this document. The project is a small, easily modified test bed for short sample routines. All of the relevant sample code is in one file: SampleCode.cs. You may need to regenerate the ..\bookdemos\WizardCode.cs file, which contains proxy classes for the Sample package (see “Generating Caché Proxy Classes” for detailed instructions).
-
console — A console program that demonstrates the bare minimum requirements for a Caché .NET project.
-
mobiledevice — Similar to adoform, but demonstrates how the mobile version of the CacheClient assembly deals with transient connections.
-
objbind — Similar to adoform, but demonstrates how to write code that uses both ADO.NET Managed Provider classes and Caché Object Binding classes in a complementary fashion.
All of these projects use classes from the Sample package in the SAMPLES namespace. You can use Studio to examine the ObjectScript code for these classes.
Most of these samples are written only in C#. If you decide to convert a sample to Visual Basic, bear in mind that a new Visual Basic .NET project will have a default namespace that contains every class defined by the project. If this is ignored, code such as:
Dim p As New Sample.Person
p = p.OpenId(CacheConnection, "1")
will fail because the root namespace has not been referenced. This can be easily corrected by disabling the "Root namespace" option in the Visual Studio project preferences.