The Caché Java Binding
The Caché Java binding provides a simple, direct way to use Caché objects within a Java application. You can create Java applications that work with the Caché database in the following ways:
-
The Caché Java Binding — The Caché Java binding lets Java applications work directly with objects on a Caché server. The binding automatically creates Java proxy classes for the Caché classes you specify. Each proxy class is a pure Java class, containing only standard Java code that provides your Java application with access to the properties and methods of the corresponding Caché class.
The Caché Java binding offers complete support for object database persistence, including concurrency and transaction control. In addition, there is a sophisticated data caching scheme to minimize network traffic when the Caché server and the Java environment are located on separate machines. This mechanism requires no object-relational mapping or additional middleware.
-
The Caché JDBC Driver — Caché includes a level 4 (pure Java) JDBC driver that supports the JDBC version 4.1 API. The Caché JDBC Driver provides high-performance relational access to Caché. For maximum flexibility, applications can use JDBC and the Caché Java binding at the same time. See Using Caché with JDBC for details.
This document assumes a prior understanding of Java and the Java standard library. Caché does not include a Java compiler or development environment.
Java Binding Architecture
The Caché Java binding gives Java applications a way to access and manipulate objects contained within a Caché server. These objects can be persistent objects stored within the Caché database or they can be transient objects that perform operations within a Caché server.
The Caché Java binding consists of the following components:
-
The Caché Java Class Generator — an extension to the class compiler that generates pure Java classes from classes defined in the Caché Class Dictionary.
-
The Caché Java Class Packages — pure Java classes that work in conjunction with the Java classes generated by the Caché Java Class Generator, providing them with transparent connectivity to the objects stored in the Caché database. (See “The Caché Java Class Packages”).
-
The Caché Object Server — a high performance server process that manages communication between Java clients and a Caché database server. It communicates using standard networking protocols (TCP/IP), and can run on any platform supported by Caché. The Caché Object Server is used by all Caché language bindings, including Java, JDBC, ODBC, C++, Perl, and Python.
The class compiler can automatically create Java client classes for any classes contained within the Caché Class Dictionary. These generated Java classes communicate at runtime with their corresponding Caché class on a Caché server. The generated Java classes contain only pure Java code and are automatically synchronized with the master class definition. This is illustrated in the following diagram:
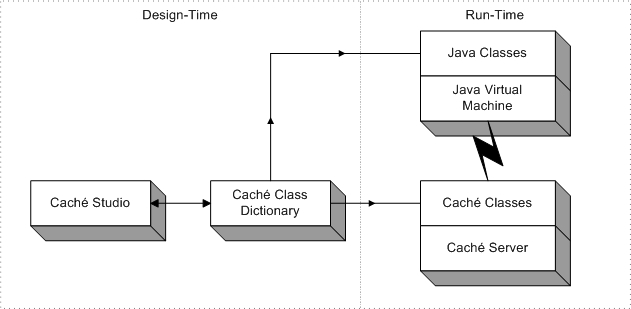
The basic mechanism works as follows:
-
You define one or more classes within Caché. These classes can represent persistent objects stored within the Caché database or transient objects that run within a Caché server.
-
The system generates Java classes that correspond to your Caché classes. These Java classes include methods which correspond to Caché methods on the server as well as accessor (get and set) methods for object properties.
-
At runtime, your Java application connects to a Caché server. It can then create instances of Java objects that correspond to objects within the Caché server. You can use these objects as you would any other Java objects. Caché automatically manages all communications as well as client-side data caching.
The runtime architecture consists of the following:
-
A Caché database server (or servers).
-
A Java Virtual Machine (JVM) in which your Java application runs. Caché does not provide a JVM but works with a standard JVM. See Java Downloads for All Operating Systems at: http://Java.com/en/download/manual.jsp for information on obtaining the Java Runtime Environment for your platform.
-
A Java application (including servlet, applets, or Swing-based applications).
-
At runtime, the Java application connects to Caché using either an object connection interface or a standard JDBC interface. All communications between the Java application and the Caché server use the TCP/IP protocol.
Installation and Configuration
All applications using the Caché Java binding are divided into two parts: a Caché server and a Java client. The Caché server is responsible for database operations as well as the execution of Caché object methods. The Java client is responsible for the execution of all Java code (such as additional business logic or the user interface). When an application runs, the Java client connects to and communicates with a Caché server via a TCP/IP socket. The actual deployment configuration is up to the application developer: the Java client and Caché server may reside on the same physical machine or they may be located on different machines. Only the Caché server machine requires a copy of Caché.
Java Client Requirements
The online InterSystems Supported PlatformsOpens in a new tab document for this release specifies the current requirements for all Java-based binding applications:
-
See “Supported Java Technologies” for supported Java releases.
-
See “Supported Client Platforms” for supported Java client platforms.
-
If your client application and the Caché server are not running on the same version of Caché, see “Supported Version Interoperability” for information on compatibility between versions.
The core components of the Java binding are files named cache-jdbc-2.0.0.jar and cache-db-2.0.0.jar, which contain the Java classes that provide the connection and caching mechanisms for communication with the Caché server, JDBC connectivity, and reflection support. Client applications do not require a local copy of Caché, but the cache-jdbc-2.0.0.jar and cache-db-2.0.0.jar files must be on the class path of the application when compiling or using Java proxy classes. See “The Caché Java Class Packages” for more information on these files.
Very little configuration is required to use a Java client with a Caché server. The Java sample programs provided with Caché should work with no change following a default Caché installation. This section describes the server settings that are relevant to Java and how to change them.
Every Java client that wishes to connect to a Caché server needs a URL that provides the server IP address, port number, and Caché namespace, plus a username and password.
The Java sample programs use the following connection information:
String url = "jdbc:Cache://127.0.0.1:1972/SAMPLES";
String user = "_SYSTEM";
String password = "SYS";
To run a Java or JDBC client application, make sure that your installation meets the following requirements:
-
The client must be able to access a machine that is currently running a compatible version of the Caché server (see “Supported Version Interoperability” in the online InterSystems Supported PlatformsOpens in a new tab document for this release). The client and the server can be running on the same machine.
-
Your class path must include the versions of cache-jdbc-2.0.0.jar and cache-db-2.0.0.jar that correspond to your version of the Java JDK (see “The Caché Java Class Packages”).
-
To connect to the Caché server, the client application must have the following information:
-
The IP address of the machine on which the Caché server is running. The Java sample programs use "localhost". If you want a sample program to connect to a different system you will need to change its connection string and recompile it.
-
The TCP/IP port number on which the Caché server is listening. The Java sample programs use 1972; if you want a sample program to use a different port you will need to change its connection string and recompile it.
-
A valid SQL username and password. You can manage SQL usernames and passwords on the System Administration > Security > Users page of the Management Portal. The Java sample programs use the administrator username, "_SYSTEM" and the default password of "SYS" or "sys". Typically, you will change the default password after installing the server. If you want a sample program to use a different username and password you will need to change it and recompile it.
-
The server namespace containing the classes and data that your client application will use. The Java samples connect to the SAMPLES namespace, which is pre-installed with Caché.
-
The Caché Java Class Packages
The files containing the Caché Java class packages are located in <install-dir>\Dev\java\lib\<java-release>, where <install-dir> is the root directory of your Caché installation and <java-release> corresponds to the Java JDK you are using. See “Caché Installation Directory” in the Caché Installation Guide for the location of <install-dir> on your system. For supported Java releases, see “Supported Java Technologies” in the online InterSystems Supported PlatformsOpens in a new tab document for this release.
The Java class packages are contained in the following files:
-
cache-jdbc-2.0.0.jar — all other files in this list are dependent on this file. It can be used by itself for JDBC binding applications.
-
cache-db-2.0.0.jar — required for most Java binding applications.
-
cache-extreme-2.0.0.jar — required only for Caché XEP binding applications (see Using Java with Caché XEP).
-
cache-gateway-2.0.0.jar — required for JDBC SQL Gateway applications (see “Using the Caché SQL Gateway with JDBC” in Using Caché with JDBC).
See the JavaDoc in <install-dir>\dev\java\doc\ for the latest and most complete description of these packages.
Other Documentation
The standard Caché installation provides JavaDoc and other documents describing various ways to use Java with Caché.
-
Complete JavaDoc is available for the Caché Java class cackages. The main index.html file is located in <install-dir>\dev\java\doc\ (see “Caché Installation Directory” for the location of <install-dir> on your system).
-
Using Caché with JDBC describes how to use the Caché JDBC driver to connect to external JDBC data sources.