Client Side Library
The chapter “Client Side Layout Managers” describes the default client side layout functionality built into Zen. Zen also offers a client side library module that allows you to override Zen defaults to provide extremely fine control over all client side activities for your application.
When your Zen page uses components that this book explicitly identifies as client side components, all entities in the client side library module are available to you automatically. For the list of these components, see the previous two chapters, “Client Side Layout Managers” and “Client Side Menu Components.” When this is the case there is no need to add any statements to your Zen page classes in order to call functions from the client side library. In other cases you can reference the library by adding it to the list of JavaScript files in the value of the JSINCLUDES parameter for the page class or application class. For example:
Parameter JSINCLUDES = "zenCSLM.js";
This chapter provides a reference guide to the JavaScript methods and properties in the Zen client side library module. This chapter documents the support that the library provides for:
By convention, the names of entities defined in the client side library use the prefix ZLM followed by a dot, as in ZLM.setDragAvatar or ZLM.getDragInnerDestination. You can think of the ZLM prefix as a namespace that keeps the library entities distinct from other functions that you might define when working with Zen.
Because the library is written in JavaScript, which does not facilitate generated class documentation, there is no Class Reference documentation for it in the InterSystems online documentation system. Refer to this chapter for all details.
Writing Custom Drag and Drop Methods
This section describes parts of the library that are important when you are customizing drag and drop methods for custom components, as outlined in the “Data Drag and Drop Methods” section in the chapter “Custom Components.”
The following table describes the methods and properties of the JavaScript object called zenDragData that is automatically made available to subclasses of %ZEN.Component.componentOpens in a new tab as a special variable called dataDrag.
Member | Meaning |
---|---|
dataDrag.toString() | This function returns a string that is equal to the value of the dataDrag.text property, if defined, otherwise the dataDrag.value property. |
dataDrag.value | The logical value of the “source” control, which is the control whose value is being dragged. |
dataDrag.text | The display value of the source control. If this is not available, the logical value is used. |
dataDrag.sourceComponent | A pointer to the source control. |
dataDrag.targetComponent | The logical value of the “target” control, which is the control onto which the value is being dropped. |
dataDrag.sourceItem | A pointer to a specific data field within the source control. For example, if the control is a list box it may contain several list entries. Only one of these entries provides the data item that is being dragged. |
dataDrag.targetItem | A pointer to a specific data field within the target control. This is the field onto which the data item is being dropped. For example, if the control is a list box this identifies a specific item within the list. |
The Zen client side library module provides the following functions to support custom drag and drop methods.
ZLM.getDragData
ZLM.getDragData()
Returns a pointer to the dragData JavaScript object whose structure is described in the section “Data Drag and Drop Methods.” This object contains all the information needed to execute a drag and drop operation.
ZLM.getDragDestination
ZLM.getDragDestination()
Returns a pointer to the enclosing <div> for the Zen element where the current drag operation ended.
ZLM.getDragInnerSource
ZLM.getDragInnerSource()
Returns a pointer to the innermost enclosing <div> element that initiated the current drag operation. This <div> is a descendant of the enclosing <div> for the Zen element that would be returned by ZLM.getDragSource.
ZLM.getDragInnerDestination
ZLM.getDragInnerDestination()
Returns a pointer to the innermost enclosing <div> element where the current drag operation ended. This <div> is a descendant of the enclosing <div> for the Zen element that would be returned by ZLM.getDragDestination.
ZLM.getDragSource
ZLM.getDragSource()
Returns a pointer to the enclosing <div> for the Zen element that initiated the current drag operation.
ZLM.setDragAvatar
ZLM.setDragAvatar(newDiv)
Allows a user-defined dragStartHandler to set the icon to use as the drag avatar of the object being dragged. The newDiv argument is the pointer to an enclosing <div> object, which can be set up for the call to ZLM.setDragAvatar as shown in the following example, and in other examples in the section “Data Drag and Drop Methods”:
Method dragStartHandler(dragData) [ Language = javascript ]
{
// get drag data
if (!this.getDragData(dragData)) {
return false;
}
// avatar
var icon = this.getEnclosingDiv().cloneNode(true);
icon.style.position="absolute";
icon.style.border ="1px solid darkgray";
icon.style.background ="#D0D0F0";
ZLM.setDragAvatar(icon);
return true;
}
ZLM.setDragCaption
ZLM.setDragCaption(caption)
Allows a user-defined dragStartHandler to set the caption string of the object being dragged. The caption argument is a text string.
Debugging Client Side Code
This topic describes a set of Zen client side library module functions that support debugging by logging messages to a console window devoted to Zen client side activities. The following is a sample message console window. The output in this example reflects calls to ZLM.cerr and ZLM.dumpObj. These are only two of the available functions.
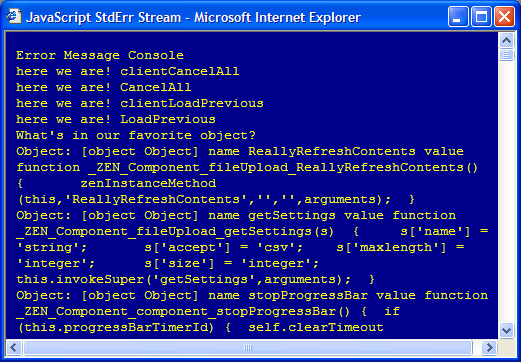
If a message console window is not already open as a result of a previous call to ZLM.showMsgConsole, any of the logging functions described in this section has the effect of opening the message console window. If there is a message console window already open, the logging function keeps writing to that window.
The logging functions are extremely useful in that they can be called from client-side JavaScript methods and from server-side Zen methods using &js<> syntax; that is:
&js<ZLM.cerr("We are now in a ZenMethod");>
This way, activities on both the server and the client side can write to the same message console window, showing the actual sequence of activities on both sides. The window stays open until full control returns to the server, for example when a form is submitted and its server-only method %OnSubmit executes. At that time the window automatically closes.
The Zen client side library module provides the following logging functions.
ZLM.showMsgConsole
ZLM.showMsgConsole()
Opens an extra window to serve as an error console. Initializes this window to respect whitespace and to use a monospaced font. As a debugging tool, this window is a useful alternative to a series of JavaScript alert calls which require you to repeatedly dismiss popup windows when messages display. The window stays open and displays all text provided by subsequent calls to ZLM.cerr.
ZLM.cerr
ZLM.cerr(msg)
Output a string to the message console window for debugging purposes. The msg argument is a text string. The following line can appear in a client-side JavaScript method:
ZLM.cerr("We are now in a client side method");
ZLM.dumpObj
ZLM.dumpObj(obj)
Write all known properties of a given object, as well as their current values, to the message console window.
The input argument obj can be any valid JavaScript object, including a Zen component or a JavaScript object such as an array, function, or custom data structure. The advantage of using ZLM.dumpObj to view the contents of an array is that you do not need to set up any iteration over the array. Simply input the array object to ZLM.dumpObj.
obj can identify a component on the Zen page, or the page itself. You can retrieve a valid obj value for a component with the client-side function zen and the id specified in the component definition. For example, if you have:
<button id="myFavoriteObject" />
Then you can dump its current properties as follows:
ZLM.cerr("What's in my favorite object?");
ZLM.dumpObj(zen("myFavoriteObject"));
Alternatively, obj can be a pointer to the enclosing <div> for the Zen element whose contents are of interest. You can retrieve this value by first invoking the client-side function zen with the id specified in the component definition, and then invoking the client-side component method getEnclosingDiv. The result is a value that you can input to the ZLM.dumpObj function. For example:
var comp = zen("thatComponent");
var div = comp.getEnclosingDiv();
ZLM.dumpObj(div);
ZLM.dumpDOMTreeGeometry
ZLM.dumpDOMTreeGeometry(root)
Write the nesting structure and base geometry of a tree of DOM nodes to the message console window. root is the root of the tree that you wish to display. root can be any valid DOM node from a JavaScript perspective. This would be any part of the HTML source that is associated with an HTML tag such as <div>, <span>, <input>, <p>, etc. — basically the web building blocks for drawing the HTML output using the component's %DrawHTML method.
To display the entire DOM tree, use document.body as the root; for example:
ZLM.cerr("What does the DOM for the entire page look like right now?");
ZLM.dumpDOMTreeGeometry(document.body);
Alternatively, root can be a pointer to the enclosing <div> for the Zen component whose DOM structure is of interest. You can retrieve this value by first invoking the client-side function zen with the id specified in the component definition, and then invoking the client-side component method getEnclosingDiv. The result is a value that you can input to the ZLM.dumpDOMTreeGeometry function. For example:
var comp = zen("secondGroupFromLeft");
var div = comp.getEnclosingDiv();
ZLM.dumpDOMTreeGeometry(div);
The call to getEnclosingDiv returns an HTML <div> element that we use as a wrapper around Zen components that might have multiple DOM nodes associated with them. This convention is designed to make sure that the DOM tree branches off in an orderly fashion. If the return value is used as input to ZLM.dumpDOMTreeGeometry you get a snapshot of the HTML structure created by the call to %DrawHTML for this component, plus any modifications to that structure resulting from applied stylesheets and/or "onload" client-side post-processing.
ZLM.dumpElementStyle
ZLM.dumpElementStyle(div)
Report all known style properties of a given component to the message console window. div is a pointer to the enclosing <div> for the Zen component whose style properties are of interest.
You can retrieve the value of div by first invoking the client-side function zen with the id specified in the component definition, and then invoking the client-side component method getEnclosingDiv. The result is a value that you can input to the ZLM.dumpElementStyle function. For example:
var comp = zen("advancedOptions");
var div = comp.getEnclosingDiv();
ZLM.dumpElementStyle(div);