Defining Custom Actions
This page describes how to define custom actions for use in dashboards, as part of the Business Intelligence implementation process.
Introduction
You define custom actions within KPI classes. Then:
-
When you display a given KPI in a widget, you can add controls to that widget that invoke the custom actions. See Adding Widget Controls.
-
If you specify a KPI class as the actionClass attribute of the <cube> element, all actions within this class are available to pivot tables that use this cube, which means they can be added as controls to widgets that display these pivot tables.
-
If you specify a KPI class as the actionClass attribute of another <kpi> element, all actions within this class are available to that KPI, in addition to any actions defined within that KPI.
-
You can execute actions from within the Analyzer. Note that in this case, only a subset of the client-side commands are supported: alert, navigate, and newWindow. Other commands are ignored.
For details on defining KPIs, see Advanced Modeling for InterSystems Business Intelligence.
You can perform many of the same operations with either a standard action or a custom action:
Operation | Available As Standard Action? | Can Be Performed in Custom Action? |
---|---|---|
Setting a filter | Yes | Yes |
Setting a filter and refreshing the display | Yes | Yes |
Refreshing the display of a widget | Yes | Yes |
Refreshing the display of the entire dashboard | Yes | No |
Specifying the row or column sort for a pivot table | Yes | No |
Specifying the row or column count for a pivot table | Yes | No |
Displaying a listing for a pivot table | Yes | No |
Displaying another dashboard | Yes | Yes |
Displaying a URL in the same page | Yes | Yes |
Displaying a URL in a new page | No | Yes |
Executing code on the server | No | Yes |
Changing the data source of the widget | Yes | No |
Changing the row or column specification of the widget | Yes | No |
For details on the standard actions, see Adding Widget Controls.
Context Information
The system makes context information available to actions, by two different mechanisms. When a user launches a custom action, the system writes context information into the pContext variable, which is available in your custom code on the server. When a custom action opens a URL, the system replaces the $$$VALUELIST and $$$CURRVALUE tokens, if these are included in the URL. The following figure illustrates these mechanisms:
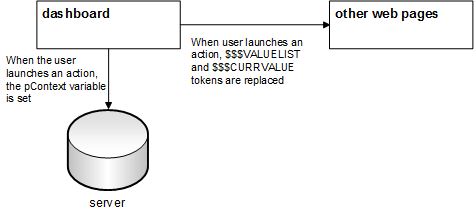
Defining the Behavior of Actions
To define custom actions, you must both declare the actions and define their behavior.
Declaring Actions
To declare actions, do either or both of the following tasks in a KPI class:
-
Within the <kpi> element, include one <action> element for each action.
This element specifies the name of an action available within this KPI class; the user interfaces use this information to create lists of available actions for the users. For example:
<kpi xmlns="http://www.intersystems.com/deepsee/kpi" name="Holefoods Actions"> <action name="ActionA"/> <action name="ActionB"/> <action name="ActionC"/> </kpi>
For information on <action>, see Reference Information for KPI and Plug-in Classes.
-
Override the %OnGetActionList() callback method of your KPI class. This method has the following signature:
ClassMethod %OnGetActionList(ByRef pActions As %List, pDataSourceName As %String = "") As %Status
Where pActions is an array with the following nodes:
Node Value pActions Number of actions pActions(n) Details for the nth action. This is a $LISTBUILD list that consists of the following items: -
A string that equals the logical action name
-
A string that equals the corresponding display name
And pDataSourceName is for future use.
For example:
ClassMethod %OnGetActionList(ByRef pActions As %List, pDataSourceName As %String = "") As %Status { set newaction=$LB("New Action","New Action Display Name") set pActions($I(pFilters))=newaction quit $$$OK }
-
Defining the Behavior of the Actions
To define the behavior of the actions, override the %OnDashboardAction() callback method of your KPI class. This method has the following signature:
classmethod %OnDashboardAction(pAction As %String, pContext As %ZEN.proxyObject) as %Status
The system executes this callback when a user invokes an action on a dashboard. pAction is the logical name of the action. pContext is an object that contains information about the currently selected scorecard row and that provides a way for the method to return commands to the dashboard; the next sections give the details.
A simple example is as follows:
ClassMethod %OnDashboardAction(pAction As %String, pContext As %ZEN.proxyObject) As %Status
{
Set sc = $$$OK
Try {
If (pAction = "Action 1") {
//this part defines Action 1
//perform server-side actions
}
Elseif (pAction="Action 2")
{
//this part defines Action 2
//perform other server-side actions
}
}
Catch(ex) {
Set sc = ex.AsStatus()
}
Quit sc
}
This method defines two actions, Action 1 and Action 2.
Because %OnDashboardAction() is a class method, you do not have access to %seriesNames or other properties of the KPI class from within this method (no class instance is available from the method).
Available Context Information
An action can use context information — values from the dashboard, based on the row or rows that the user selected before launching the action. These values are useful if you want to cause changes in the database that are dependent on context.
Because %OnDashboardAction() is a class method, you do not have access to %seriesNames or other properties of the KPI class from within this method. Instead, the system provides the pContext variable, which is an object whose properties provide information for use in the action. The details are different in the following scenarios:
-
Pivot table widget that uses a pivot table as the data source
-
Scorecard that uses either a pivot table or a KPI as the data source
Scenario: Pivot Table Widget with Pivot Table as Data Source
In this scenario, within the %OnDashboardAction() method, the pContext object contains the properties described in the following table. As noted, the contents of the pContext object vary depending on whether the widget is displaying the pivot table itself (pivot mode) or a listing (listing mode).
Property Name | Contents in Pivot Mode | Contents in Listing Mode |
---|---|---|
currValue | Value of first selected cell | Value of the first selected cell that was displayed before the listing was shown |
currSeriesNo | Column number | Column number of the first selected cell that was displayed before the listing was shown |
currItemNo | Row number | Null |
currFilterSpec | MDX %FILTER clause or clauses that represent the filtering applied to the current cell context. This includes values of any filter controls, as well as the row and column context. | Null |
valueList | Null | Comma-separated list of values from the first column of the listing (these values must not contain commas) |
cubeName | Name of the cube queried by this pivot table | Null |
mdx | MDX query defined by this pivot table | Null |
pivotVariables | A proxy object that contains one property for each pivot variable. Specifically, pContext.pivotVariables.varname contains the value of the pivot variable varname. In this proxy object, all pivot variable names are in lowercase. For example, if the server defines a pivot variable named MyVar, this pivot variable is available as pContext.pivotVariables.myvar | Same as in pivot mode |
filters | An array that indicates the current values of the filter controls which are currently active. The subscript for each node in the array is the MDX expression for the filter. The value of the node is the corresponding key or keys, in the form described at Allowed Default Values for Filters. | Same as in pivot mode |
dataSource | Name of the current data source | Name of the current data source |
Scenario: Pivot Table Widget with KPI as Data Source
In this scenario, within the %OnDashboardAction() method, the pContext object contains the properties described in the following table. As noted, the contents of the pContext object vary depending on whether the widget is displaying the pivot table itself (pivot mode) or a listing (listing mode).
Property Name | Contents in Pivot Mode | Contents in Listing Mode |
---|---|---|
currValue | Value of first selected cell | Value of the first selected cell that was displayed before the listing was shown |
currSeriesNo | Column number | Column number of the first selected cell that was displayed before the listing was shown |
valueList | Null | Comma-separated list of values from the first column of the listing (these values must not contain commas) |
pivotVariables | A proxy object that contains one property for each pivot variable. Specifically, pContext.pivotVariables.varname contains the value of the pivot variable varname. In this proxy object, all pivot variable names are in lowercase. For example, if the server defines a pivot variable named MyVar, this pivot variable is available as pContext.pivotVariables.myvar | Same as in pivot mode |
filters | An array that indicates the current values of all filter controls. Each node in the array corresponds to one of the filters defined by the data source KPI. The subscript for each node in the array is the name of the filter, as specified in the KPI definition class. The value of the node is the key or keys currently selected for that filter, in the form described in Allowed Default Values for Filters. If no keys are selected for a filter, the value of the corresponding node is null. | Same as in pivot mode |
dataSource | Name of the current data source | Name of the current data source |
Scenario: Scorecard with Pivot Table or KPI as Data Source
For scorecards, the contents of the pContext object within the %OnDashboardAction() method are mostly the same regardless of whether the data source is a pivot table or a KPI. The following table describes the contents of pContext for a scorecard, noting where there are variations depending on the data source:
Property Name | Contents with Pivot Table as Data Source | Contents with KPI as Data Source |
---|---|---|
currValue | Value of the pivot column that is marked as Value Column in this scorecard | Value of the KPI property that is marked as Value Column in this scorecard |
currSeriesNo | Row number | Same as when the data source is a pivot table |
valueList | Value of the pivot column that is marked as Value Column in this scorecard | Value of the KPI property that is marked as Value Column in this scorecard |
pivotVariables | A proxy object that contains one property for each pivot variable. Specifically, pContext.pivotVariables.varname contains the value of the pivot variable varname. In this proxy object, all pivot variable names are in lowercase. For example, if the server defines a pivot variable named MyVar, this pivot variable is available as pContext.pivotVariables.myvar | Same as when the data source is a pivot table |
filters | An array that indicates the current values of the filter controls which are currently active. The subscript for each node in the array is the MDX expression for the filter. The value of the node is the corresponding key or keys, in the form described at Allowed Default Values for Filters. | An array that indicates the current values of all filter controls. Each node in the array corresponds to one of the filters defined by the data source KPI. The subscript for each node in the array is the name of the filter, as specified in the KPI definition class. The value of the node is the key or keys currently selected for that filter, in the form described in Allowed Default Values for Filters. If no keys are selected for a filter, the value of the corresponding node is null. |
dataSource | Name of the current data source | Same as when the data source is a pivot table |
Executing Client-Side Commands
An action can contain commands to execute when the control returns to the dashboard. To include such commands, set the pContext.command property within the definition of the action. For example:
//this part defines Action 1
//perform server-side actions
//on returning, refresh the widget that is using this KPI
Set pContext.command="refresh;"
For pContext.command, specify a string of the following form to execute a single command:
command1
For pContext.command, specify a semicolon-delimited list of commands:
command1;command2;command3;...;
The final semicolon is optional.
Some commands accept one or more arguments. For these, use command:arg1:arg2:... instead of command.
Available Commands
Within pContext.command, you can use the following commands:
alert:message
Displays the message in a dialog box. message is the message to display
For example:
Set pContext.command = "alert:hello world!"
applyFilter:target:filterSpec
For information on the arguments, see Details for applyFilter and setFilter.
This command sets the given filter and refreshes the browser window.
For example, the following applies a filter to a pivot table:
Set pContext.command = "applyFilter:samplepivot:[DateOfSale].[Actual].[YearSold]:&[2008]"
navigate:url
Where url is the URL to display.
This command opens the given URL in the same browser window.
For example:
Set pContext.command = "navigate:http://www.google.com"
newWindow:url
Where url is the URL to display.
This command opens the given URL in a new browser window.
For example:
Set pContext.command = "newWindow:http://www.google.com"
popup:popupurl
Where popupurl is the relative URL of a popup window.
This command displays the given popup window. For example:
Set pContext.command = "popup:%ZEN.Dialog.fileSelect.cls"
refresh:widgetname
Where widgetname is the optional name of a widget to refresh; if you omit this argument, the command refreshes the widget from which the user launched the action.
This refreshes the browser window, using any current settings for filters.
For example:
// Refresh the widget that fired this action and another named samplepivot.
Set pContext.command = "refresh;refresh:samplepivot"
Note that this example includes multiple commands, separated by a semicolon.
setFilter:target:filterSpec
For information on the arguments, see Details for applyFilter and setFilter.
This command sets the given filter, but does not refresh the browser window.
Details for applyFilter and setFilter
The applyFilter and setFilter commands are as follows, respectively:
applyFilter:target:filterSpec
And:
setFilter:target:filterSpec
Where:
-
target is the widget to filter. You can use an asterisk (*) to apply the filter to all widgets.
-
filterSpec specifies the filter value or values to apply to the given target. This must have the following form:
filter_name:filter_values
Where the arguments depend upon the details of the target widget as follows:
Scenario filter_name filter_values Target widget displays a pivot table [dimension].[hierarchy].[level] See Allowed Default Values for Filters in Configuring Settings. Target widget displays a KPI Name of a filter defined in that KPI One of the allowed values for this filter, as defined in the KPI Notes:
-
You can use multiple filter specifications; to do so, separate them with a tilde (~). For example:
FILTER:filterspec1~filterspec2
-
The filter name and filter values are not case-sensitive for pivot tables or for KPIs that use MDX queries.
-
The filter can affect only widgets that have been configured with a filter control (possibly hidden) that uses the same filter. For example, suppose that a widget includes a Cities filter control, and has no other filter controls. If the action filters to a city, the widget is updated. If the action filters to a ZIP code, the widget is not updated.
-
Displaying a Different Dashboard
In your custom action, you can use navigate or newWindow to display a different dashboard. Use the dashboard URL as described in Accessing Dashboards from Your Application. The URL can include the SETTINGS keyword, which initializes the state of the dashboard.
Generating a SQL Table from Cube Context
In your custom action, you can use the %CreateTable API to create a SQL table from cube context. The table may be created from either:
-
A field list
-
The name of a listing defined in the cube, either as a field list or a custom SQL query.
See the class referenceOpens in a new tab for more details.