$Order Loop
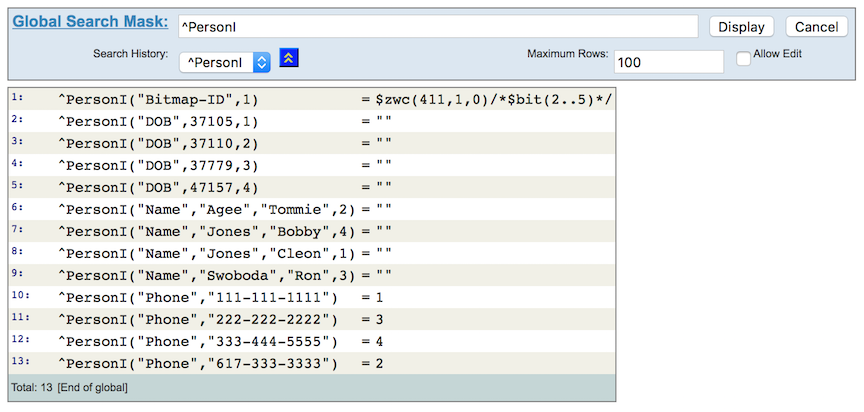
From the example on the previous page, you can see that traversing a tree involves using $Order repeatedly. Let's use a loop to make it easier, and traverse another level of the tree: last names.
The examples show two different ways to accomplish the same thing. The comments in the code explain the steps. Notice that although there are four people in the database, the loop only produces the three unique last names; if you picture the nodes in the tree, this makes sense. Also notice how neither loop produces the phone numbers, even though they appear right after the names, because the nodes for phone numbers are not siblings of the nodes for names.
The Set statement within the loops using $Order is similar to typical programming statements like set i = i + 1, using the current value of a variable to produce a new value.
/// examples for ObjectScript Tutorial
Class ObjectScript.Examples
{
/// loop through last names of the ^PersonI global, 2 different ways
ClassMethod SimpleLoop()
{
write !, "Using argumentless For"
set ln = "" // initialize to the empty string to make $Order return the first last name
for { // start looping
set ln = $order(^PersonI("Name", ln)) // use the current last name to get the next
quit:(ln = "") // stop looping when ln becomes empty again
write !, ?5, ln
}
write !!, "Using While"
set ln = $order(^PersonI("Name", "")) // get the first last name
while (ln '= "") { // only loop if there is at least one last name
write !, ?5, ln
set ln = $order(^PersonI("Name", ln)) // use the current last name to get the next
}
}
}
USER>do ##class(ObjectScript.Examples).SimpleLoop()
Using argumentless For
Agee
Jones
Swoboda
Using While
Agee
Jones
Swoboda
USER>