Using the FTP Inbound Adapter
This page describes how to use the FTP inbound adapter (EnsLib.FTP.InboundAdapterOpens in a new tab), which can provide both FTP and SFTP input to a production.
InterSystems IRIS® data platform also provides specialized business service classes that use this adapter, and one of those might be suitable for your needs. If so, no programming would be needed. See Connectivity Options.
Overall Behavior
The EnsLib.FTP.InboundAdapterOpens in a new tab enables InterSystems IRIS to receive files via the FTP protocol. The adapter receives FTP input from the configured location, reads the input, and sends the input as a stream to the associated business service. The business service, which you create and configure, uses this stream and communicates with the rest of the production.
If the FTP server expects an acknowledgment or response to its input, the business service is also responsible for formulating this response and relaying it back to the data source, via the EnsLib.FTP.InboundAdapterOpens in a new tab. The adapter does not evaluate or supplement the response, but relays it, if it is provided.
The following figure shows the overall flow of inbound messages (but not the responses):
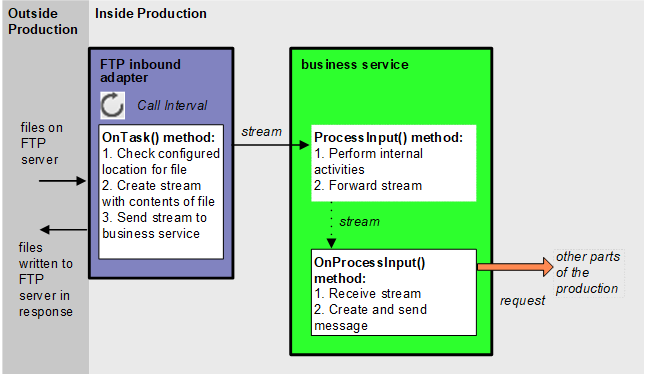
In more detail:
-
Each time the adapter encounters input from its configured data source, it calls the internal ProcessInput() method of the business service class, passing the stream as an input argument.
-
The internal ProcessInput() method of the business service class executes. This method performs basic production tasks such as maintaining internal information as needed by all business services. You do not customize or override this method, which your business service class inherits.
-
The ProcessInput() method then calls your custom OnProcessInput() method, passing the stream object as input. The requirements for this method are described in Implementing the OnProcessInput() Method.
If the data source expects an acknowledgment or response of some kind, the OnProcessInput() method of the business service creates it. The inbound adapter simply relays this response back to the external data source.
The response message follows the same path, in reverse.
Creating a Business Service to Use the Inbound Adapter
To use this adapter in your production, create a new business service class as described here. Later, add it to your production and configure it. You must also create appropriate message classes, if none yet exist. See Defining Messages.
The following list describes the basic requirements of the business service class:
-
Your business service class should extend Ens.BusinessServiceOpens in a new tab.
-
In your class, the ADAPTER parameter should equal EnsLib.FTP.InboundAdapterOpens in a new tab.
-
Your class should implement the OnProcessInput() method, as described in Implementing the OnProcessInput Method
-
For other options and general information, see Defining a Business Service Class and Example Business Service Class.
Implementing the OnProcessInput() Method
Within your custom business service class, your OnProcessInput() method should have the following signature:
Method OnProcessInput(pInput As %CharacterStream,pOutput As %RegisteredObject) As %Status
Or:
Method OnProcessInput(pInput As %BinaryStream,pOutput As %RegisteredObject) As %Status
Where:
-
pInput is the message object that the adapter will send to this business service. This can be of type %CharacterStreamOpens in a new tab or %BinaryStreamOpens in a new tab, depending on the contents of the expected stream. You use an adapter setting (Charset) to indicate whether the input stream is character or binary; see Reference for Settings.
-
pOutput is the generic output argument required in the method signature.
The OnProcessInput() method should do some or all of the following:
-
Examine the input stream (pInput) and decide how to use it.
This input type depends on the value of the Charset adapter setting:
-
When the Charset setting has the value Binary, pInput is of type %BinaryStreamOpens in a new tab and contains bytes.
-
Otherwise, pInput is of type %CharacterStream Opens in a new tab and contains characters.
For details about Charset, see Reference for Settings.
-
-
Create an instance of the request message, which will be the message that your business service sends.
For information on creating message classes, see Defining Messages.
-
For the request message, set its properties as appropriate, using values in the input.
-
Call a suitable method of the business service to send the request to some destination within the production. Specifically, call SendRequestSync(), SendRequestAsync(), or (less common) SendDeferredResponse(). For details, see Sending Request Messages.
Each of these methods returns a status (specifically, an instance of %StatusOpens in a new tab).
-
Make sure that you set the output argument (pOutput). Typically you set this equal to the response message that you have received. This step is required.
-
Return an appropriate status. This step is required.
Invoking Adapter Methods
Within your business service, you might want to invoke the following instance methods of the adapter:
Method Connect(pTimeout As %Numeric = 30,
pInbound As %Boolean = 0) As %Status
Connect to the FTP server and log in, setting the directory and transfer mode.
Method Disconnect(pInbound As %Boolean = 0) As %Status
Disconnect from the FTP server.
Method ParseFilename(pFilenameLine As %String,
Output pTimestamp As %String,
Output pSize As %String) As %Boolean
Method TestConnection()
Correct the properties reflecting the connection state, in case the adapter thinks it is connected but has lost the socket.
The following methods are also available. Each method corresponds to an adapter setting that the user can adjust using the Management Portal. Each time the user clicks Apply to accept changes to the value of a Setting, the corresponding SettingSet method executes. These methods provide the opportunity to make adjustments following a change in any setting. For detailed descriptions of each setting, see Reference for Settings.
Method ArchivePathSet(pInVal As %String) As %Status
ArchivePath is the directory where the adapter should place a copy of each file after processing.
Method CharsetSet(cset As %String) As %Status
Charset is the character set of the input file.
Method ConnectedSet(pValue As %Boolean) As %Status
Connected is an internal property that tracks the adapter’s connection to the FTP server.
Method CredentialsSet(pInVal As %String) As %Status
Credentials is a production credentials entry that can authorize a connection to the FTP server. For information on creating production credentials, see Defining Credentials.
Method FilePathSet(path As %String) As %Status
FilePath is the directory on the FTP server in which to look for files.
Method FTPPortSet(port As %String) As %Status
FTPPort is the TCP Port on the FTP Server to connect to.
Method FTPServerSet(server As %String) As %Status
FTPServer is the FTP Server to connect to. This can be the IP address or server name, as long as the domain host controller can resolve the name.
Method SSLConfigSet(sslcfg As %String) As %Status
SSLConfig is the TLS configuration entry to use to authenticate this connection.
Example Business Service Class
The following code example shows a business service class that references the EnsLib.FTP.InboundAdapterOpens in a new tab.
Class EnsLib.FTP.PassthroughService Extends Ens.BusinessService
{
Parameter ADAPTER = "EnsLib.FTP.InboundAdapter";
/// Configuration item(s) to which to send file stream messages
Property TargetConfigNames As %String(MAXLEN = 1000);
Parameter SETTINGS = "TargetConfigNames";
/// Wrap the input stream object in a StreamContainer message object and send
/// it. If the adapter has a value for ArchivePath, send async; otherwise send
/// synchronously to ensure that we don't return to the Adapter and let it
/// delete the file before the target Config Item is finished processing it.
Method OnProcessInput(pInput As %Stream.Object,
pOutput As %RegisteredObject) As %Status
{
Set tSC=$$$OK, tSource=pInput.Attributes("Filename"),
tFileLocation=pInput.Attributes("FTPDir"),
pInput=##class(Ens.StreamContainer).%New(pInput)
Set tWorkArchive=(""'=..Adapter.ArchivePath)
For iTarget=1:1:$L(..TargetConfigNames, ",") {
Set tOneTarget=$ZStrip($P(..TargetConfigNames,",",iTarget),"<>W")
Continue:""=tOneTarget
$$$sysTRACE("Sending input Stream ...")
If tWorkArchive {
Set tSC1=..SendRequestAsync(tOneTarget,pInput)
Set:$$$ISERR(tSC1) tSC=$$$ADDSC(tSC,tSC1)
} Else {
#; If not archiving send Sync to avoid Adapter deleting file before
#; Operation gets it
Set tSC1=..SendRequestSync(tOneTarget,pInput)
Set:$$$ISERR(tSC1) tSC=$$$ADDSC(tSC,tSC1)
}
}
Quit tSC
}
}
Note that this example sets two variables to capture metadata about the incoming stream, pInput:
-
tSource captures the original file name, which is stored in the Filename subscript of the Attributes property of the incoming stream
-
tFileLocation captures the complete original file path, which is stored in the FTPDir subscript of the same property
Adding and Configuring the Business Service
To add your business service to a production, use the Management Portal to do the following:
-
Add an instance of your business service class to the production.
-
Configure the business service. For information on the settings, see Reference for Settings.
-
Enable the business service.
-
Run the production.
See Also
-
Using and debugging %Net.SSH.Session for SSH connectionsOpens in a new tab (article on the Developer Community)